In today’s interconnected world, software applications often need to communicate with external services to fetch or share data. Integrating a JavaFX application with RESTful web services is a common requirement, allowing you to create powerful and dynamic user interfaces that interact with remote data sources. In this article, we will explore the integration of JavaFX and RESTful web services to build compelling desktop applications.
What are RESTful Web Services?
REST (Representational State Transfer) is an architectural style for designing networked applications. RESTful web services are web services that adhere to REST principles. They use HTTP methods like GET, POST, PUT, DELETE to perform CRUD (Create, Read, Update, Delete) operations on resources identified by URIs (Uniform Resource Identifiers).
RESTful web services are widely used for data exchange between different systems, making them a suitable choice for integrating external data sources with JavaFX applications.
Why Integrate JavaFX and RESTful Web Services?
Integrating JavaFX with RESTful web services can be incredibly beneficial for your desktop applications:
- Data Connectivity: RESTful web services allow your JavaFX application to connect to external data sources, such as databases or online APIs, to retrieve and manipulate data.
- Real-time Updates: You can easily implement real-time updates in your JavaFX application by making asynchronous HTTP requests to RESTful APIs.
- Cross-Platform Compatibility: Since JavaFX runs on multiple platforms, you can create cross-platform desktop applications that can access web services regardless of the operating system.
- Scalability: RESTful web services are inherently scalable, which means your JavaFX application can handle a growing user base and increasing data requirements without significant changes.
Integrating JavaFX and RESTful Web Services
In this tutorial, we’ll create a simple JavaFX application that consumes data from a RESTful web service and displays it in a graphical user interface. We’ll use the popular JSONPlaceholder API to fetch fake posts, and the Gson library to parse JSON responses.
Installing Gson
Gson, developed by Google, is a Java library that provides efficient JSON serialization and deserialization capabilities, making it a preferred choice for handling JSON data in Java applications. Installing Gson can be accomplished through two main methods: manually downloading the JAR from the Maven Repository or utilizing build automation tools such as Maven or Gradle. Using build automation tools is the recommended and more convenient approach. Additionally, Maven Repository provides the necessary code snippets for integrating Gson with these build tools, simplifying the installation process.
Create a Model Class
Create a Java class to represent the data structure of a post. This class will be used to deserialize the JSON response from the RESTful web service. We’ll define a Post class with fields like userId, id, title and body.
public class Post {
private int userId;
private int id;
private String title;
private String body;
public int getUserId() {
return userId;
}
public int getId() {
return id;
}
public String getTitle() {
return title;
}
public String getBody() {
return body;
}
}
Implement the RESTful API Client
You need a Java class to make HTTP requests to the RESTful web service and parse the JSON response. We’ll use the java.net.HttpURLConnection class to send GET requests and the Gson library to parse JSON responses. Here’s a simplified PostClient class:
import com.google.gson.Gson;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class PostClient {
private static final String API_URL = "https://jsonplaceholder.typicode.com/posts";
public static Post[] getAll() throws IOException {
HttpURLConnection connection = null;
try {
// Create a URL object and open a connection
URL url = new URL(API_URL);
connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
// Get the HTTP response code
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
// Use Gson to deserialize the JSON response into an array of Post objects
Gson gson = new Gson();
return gson.fromJson(parseResponse(connection), Post[].class);
} else {
// Handle API request failure with an exception
throw new IOException("API request failed with response code: " + responseCode);
}
} finally {
if (connection != null) {
connection.disconnect();
}
}
}
private static String parseResponse(HttpURLConnection connection) throws IOException {
try (BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()))) {
StringBuilder response = new StringBuilder();
String line;
// Read the response content line by line
while ((line = reader.readLine()) != null) {
response.append(line);
}
return response.toString();
}
}
}
Displaying Data
Now that we have successfully implemented the RESTful API client to fetch data from a web service, let’s integrate it with our JavaFX application to display the retrieved data in a user-friendly graphical interface.
To display the data, we’ll create a JavaFX user interface with a TableView component to present the posts in a tabular format.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.ProgressIndicator;
import javafx.scene.control.TableColumn;
import javafx.scene.control.TableView;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
// The parent layout manager
private final BorderPane parent = new BorderPane();
// Create a TableView to display the data
private final TableView<Post> tableView = new TableView<>();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
TableColumn<Post, Integer> userIdColumn = new TableColumn<>("USER ID");
TableColumn<Post, Integer> idColumn = new TableColumn<>("ID");
TableColumn<Post, String> titleColumn = new TableColumn<>("Title");
TableColumn<Post, String> bodyColumn = new TableColumn<>("Body");
// Define how the columns should retrieve data from the Post class
userIdColumn.setCellValueFactory(new PropertyValueFactory<>("userId"));
idColumn.setCellValueFactory(new PropertyValueFactory<>("id"));
titleColumn.setCellValueFactory(new PropertyValueFactory<>("title"));
bodyColumn.setCellValueFactory(new PropertyValueFactory<>("body"));
// Add columns to the TableView
this.tableView.getColumns().addAll(
userIdColumn,
idColumn,
titleColumn,
bodyColumn
);
// Initially, show a ProgressIndicator to indicate data loading
this.parent.setCenter(new ProgressIndicator());
}
@Override
public void start(Stage stage) throws Exception {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Integrating JavaFX and RESTful Web Services");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this code, we create a TableView with columns for User ID, ID, Title, and Body. We also specify how data should be retrieved from the Post class using the PropertyValueFactory. However, rather than presenting an empty table initially, we’ve implemented a ProgressIndicator to signify that data is currently being loaded within our application.
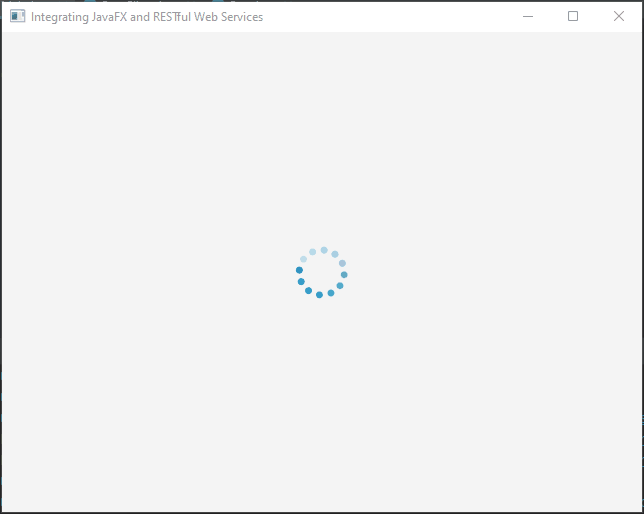
Populating the TableView with Data
Now that we have our user interface set up, let’s integrate the PostClient to fetch data from the RESTful web service and populate the TableView.
import javafx.application.Application;
import javafx.application.Platform;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.control.ProgressIndicator;
import javafx.scene.control.TableColumn;
import javafx.scene.control.TableView;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.scene.layout.*;
import javafx.stage.Stage;
import java.io.IOException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class Main extends Application {
// The parent layout manager
private final BorderPane parent = new BorderPane();
// Create a TableView to display the data
private final TableView<Post> tableView = new TableView<>();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
TableColumn<Post, Integer> userIdColumn = new TableColumn<>("USER ID");
TableColumn<Post, Integer> idColumn = new TableColumn<>("ID");
TableColumn<Post, String> titleColumn = new TableColumn<>("Title");
TableColumn<Post, String> bodyColumn = new TableColumn<>("Body");
// Define how the columns should retrieve data from the Post class
userIdColumn.setCellValueFactory(new PropertyValueFactory<>("userId"));
idColumn.setCellValueFactory(new PropertyValueFactory<>("id"));
titleColumn.setCellValueFactory(new PropertyValueFactory<>("title"));
bodyColumn.setCellValueFactory(new PropertyValueFactory<>("body"));
// Add columns to the TableView
this.tableView.getColumns().addAll(
userIdColumn,
idColumn,
titleColumn,
bodyColumn
);
// Initially, show a ProgressIndicator to indicate data loading
this.parent.setCenter(new ProgressIndicator());
}
@Override
public void start(Stage stage) throws Exception {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Fetch data in a background thread from the
// RESTful web service to keep the UI responsive
ExecutorService executor = Executors.newSingleThreadExecutor();
executor.submit(this::fetchData);
// Set the stage title
stage.setTitle("Integrating JavaFX and RESTful Web Services");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
private void fetchData() {
try {
Post[] posts = PostClient.getAll();
ObservableList<Post> postList = FXCollections.observableArrayList(posts);
// Update the UI on the JavaFX Application Thread
Platform.runLater(() -> {
this.tableView.setItems(postList);
this.parent.setCenter(this.tableView);
});
} catch (IOException e) {
// Handle the exception and display an error message to the user
e.printStackTrace();
}
}
}
In this updated code, we have set up a TableView to display data fetched from a RESTful web service using the PostClient.getAll() method. We use an ObservableList to manage the data, enabling real-time updates in the TableView as new data is received. To maintain a responsive user interface, we fetch data in a background thread using an ExecutorService.
Additionally, we’ve taken care to include error handling, which allows us to provide users with informative error messages in case any exceptions occur during the data retrieval process. This approach ensures a smooth and user-friendly experience when interacting with the application.
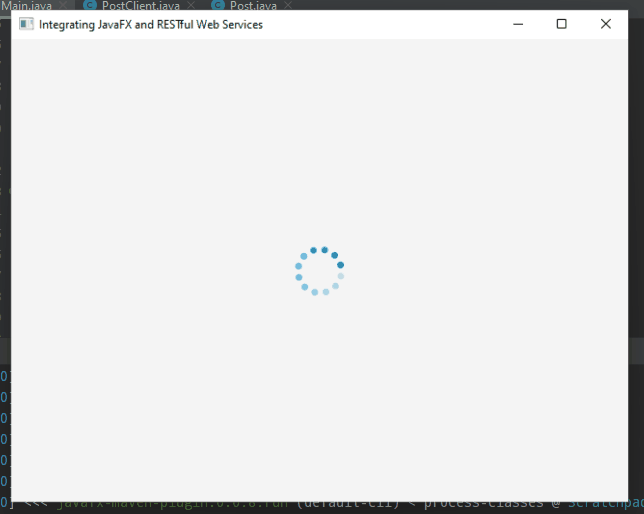
Conclusion
Integrating JavaFX and RESTful web services allows you to build powerful desktop applications that can fetch and display data from remote sources in a user-friendly manner. In this tutorial, we created a JavaFX application with a TableView to display posts retrieved from a RESTful web service.
Remember that in real-world applications, you should handle errors, perform data parsing, and ensure the user interface remains responsive during API requests. Additionally, consider using libraries like Apache HttpClient or OkHttp for more robust and efficient HTTP communication.
By mastering the integration of JavaFX and RESTful web services, you can create powerful desktop applications that provide seamless access to remote data, opening up a world of possibilities for your software projects.
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter.