QR (Quick Response) codes have become increasingly popular for various applications, from sharing URLs to storing information such as contact details, product information, and more. If you’re a Java developer working with JavaFX, you might wonder how to generate QR codes efficiently. In this article, we’ll guide you through the process of creating QR codes using JavaFX, providing you with concise and complete code examples.
Prerequisites
Before we dive into the code, make sure you have the following set up:
- Java Development Kit (JDK) installed on your machine.
- A Java Integrated Development Environment (IDE) such as Eclipse or IntelliJ IDEA.
Setting Up the Project
First, create a new JavaFX project in your IDE. Make sure you have the necessary JavaFX libraries included in your project’s classpath.
Add QR Code Generation Library
For this example, we’ll use the “ZXing” (Zebra Crossing) library, a popular QR code generation library for Java. To include it in your project, you can either download the JAR file manually or use a build tool like Maven or Gradle to manage the dependency. For simplicity, we’ll use Maven.
Add the following Maven dependency to your pom.xml file:
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>core</artifactId>
<version>3.5.2</version>
</dependency>
Once you’ve added the dependency, make sure to update the project so that the library is downloaded and available for use.
Generating the QR Code
Now that we have set up the project and added the QR code generation library, let’s proceed with the code for creating QR codes.
import com.google.zxing.BarcodeFormat;
import com.google.zxing.WriterException;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.QRCodeWriter;
import javafx.util.Builder;
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
public class QRGenerator {
private final BitMatrix bitMatrix;
private BufferedImage qrImage;
public QRGenerator(String data, BarcodeFormat format, int width, int height, int imageType) throws WriterException {
BitMatrixBuilder builder = new BitMatrixBuilder(data, format, width, height);
this.bitMatrix = builder.build();
this.qrImageCreate(width, height, imageType);
}
private void qrImageCreate(int width, int height, int imageType) {
// Convert BitMatrix to BufferedImage
this.qrImage = new BufferedImage(width, height, imageType);
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
qrImage.setRGB(x, y, this.bitMatrix.get(x, y) ? 0xFF000000 : 0xFFFFFFFF);
}
}
}
public void save(String pathname, String formatName) throws IOException {
ImageIO.write(qrImage, formatName, new File(pathname));
}
public BufferedImage qrImage() {
return this.qrImage;
}
private static class BitMatrixBuilder implements Builder<BitMatrix> {
private final BitMatrix bitMatrix;
public BitMatrixBuilder(String data, BarcodeFormat format, int width, int height) throws WriterException {
QRCodeWriter qrCodeWriter = new QRCodeWriter();
this.bitMatrix = qrCodeWriter.encode(data, format, width, height);
}
@Override
public BitMatrix build() {
return this.bitMatrix;
}
}
}
We start by implementing a helper class called QRGenerator, which encapsulates the logic for generating QR codes using the ZXing library. The class takes care of the QR code generation process and provides a way to save the generated QR code as an image file and it also provides a way to retrieve the resulting QR code as a BufferedImage. It adds a layer of abstraction by introducing an inner static class called BitMatrixBuilder, which implements the JavaFX Builder interface.
To use the QRGenerator, you would instantiate the class and call the qrImage() method to obtain the generated QR code as a BufferedImage, and the save() method to save the generated QR code as an image file.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.WriterException;
import javafx.embed.swing.SwingFXUtils;
import javafx.scene.image.ImageView;
import java.awt.image.BufferedImage;
import java.io.IOException;
public class Main extends Application {
private static final int QR_CODE_SIZE = 300;
private static final String CONTENT_TO_ENCODE = "JavaFX QR Code Generator";
private final StackPane parent = new StackPane();
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
QRGenerator qrGenerator;
try {
qrGenerator = new QRGenerator(
CONTENT_TO_ENCODE,
BarcodeFormat.QR_CODE,
QR_CODE_SIZE, QR_CODE_SIZE,
BufferedImage.TYPE_INT_ARGB
);
} catch (WriterException e) {
e.printStackTrace();
return;
}
BufferedImage qrImage = qrGenerator.qrImage();
// Save QR code as an image file (optional)
try {
qrGenerator.save("qrcode.png", "PNG");
} catch (IOException e) {
e.printStackTrace();
}
Image image = SwingFXUtils.toFXImage(qrImage, null);
// Display QR code in JavaFX window
ImageView imageView = new ImageView(image);
parent.getChildren().add(imageView);
}
@Override
public void start(Stage stage) throws Exception {
this.setupStage(stage);
}
private void setupStage(Stage stage) {
Scene scene = new Scene(this.parent, 640.0, 480.0);
// Sets the stage title
stage.setTitle("JavaFX QR Code Generator");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
}
The provided code is an implementation of a JavaFX application that generates a QR code using the QRGenerator class and displays it in a JavaFX window. It also saves the generated QR code as an image file named “qrcode.png” in the project directory.
When you run the JavaFX application, it will display a window showing the generated QR code based on the CONTENT_TO_ENCODE. The QR code will also be saved as an image file named “qrcode.png” in the project directory (make sure the program has the necessary write permissions).
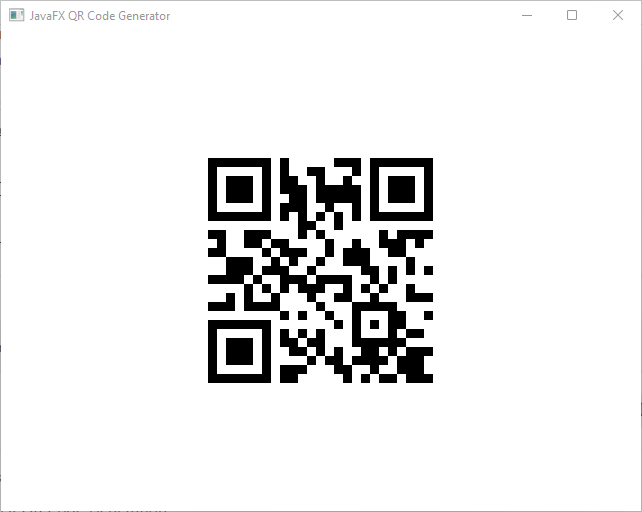
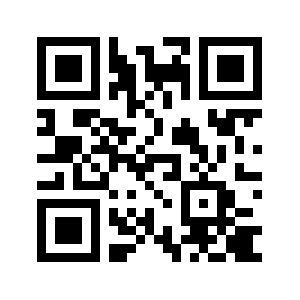
By encapsulating the QR code generation process into a separate class, the QRGenerator provides a clean and modular way to generate QR codes throughout your JavaFX application. It allows you to easily customize the QR code’s data, size, format, and image type without duplicating code or worrying about the intricate details of the QR code generation library.
Conclusion
Congratulations! You have successfully created a JavaFX application that generates QR codes using the ZXing library. QR codes have various applications, and you can integrate this code into your projects to create dynamic QR codes with different data.
Feel free to explore more options offered by the ZXing library, such as error correction, encoding different data types, and customizing QR code appearance.
I hope you found this code informative, and useful. If you would like to receive more content, please consider subscribing to our newsletter!