When working with JavaFX applications, there are often scenarios where you need to interact with the graphical elements on the screen. One common task is retrieving the color of a pixel at a specific location on the screen. This can be useful for a variety of purposes, such as creating color pickers, implementing color-based interactions, or automating tasks that depend on the screen content.
JavaFX provides a convenient way to achieve this using the javafx.scene.robot.Robot class. In this article, we will explore how to use the Robot class to retrieve the color of a pixel on the screen.
The Robot Class
The Robot class is part of the JavaFX API and is designed to interact with the graphical user interface of JavaFX applications. It allows you to simulate user input events (such as mouse clicks and key presses) and retrieve information from the screen, including pixel colors.
Retrieving Screen Pixel Colors
Retrieving the color of a pixel on the screen using the Robot class is straightforward. Here’s a simple example:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.input.MouseEvent;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.scene.robot.Robot;
import javafx.stage.Stage;
public class Main extends Application {
// The parent layout manager
private final StackPane parent = new StackPane();
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() {
// Load an image (Replace with your image path)
Image image = new Image("image.jpg");
// Create an ImageView to display the image
ImageView imageView = new ImageView(image);
// Attach click event handler
imageView.setOnMouseClicked(this::onImageViewClicked);
// Maintain aspect ratio
imageView.setPreserveRatio(true);
// Set the image width
imageView.setFitWidth(300.0);
// Add the ImageView to the parent layout (StackPane)
this.parent.getChildren().add(imageView);
}
private void onImageViewClicked(MouseEvent mouseEvent) {
// Initialize the Robot for screen pixel color retrieval
Robot robot = new Robot();
// Get the ImageView that triggered the click event
ImageView imageView = (ImageView) mouseEvent.getSource();
// Get the color of the pixel at the clicked coordinates
Color pixelColor = robot.getPixelColor(robot.getMouseX(), robot.getMouseY());
// Set the background color of the parent StackPane to the selected color
imageView.getParent().setStyle(String.format(
"-fx-background-color: rgba(%d, %d, %d, %.2f);",
(int) (pixelColor.getRed() * 255),
(int) (pixelColor.getGreen() * 255),
(int) (pixelColor.getBlue() * 255),
pixelColor.getOpacity()
));
}
@Override
public void start(Stage stage) throws Exception {
// Create a scene with the StackPane as the root
Scene scene = new Scene(parent, 640, 480);
// Set the stage title
stage.setTitle("Screen Pixel Color Retrieval in JavaFX");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this example, we created an application that displays an image, and when a user clicks on the image, the code captures the color of the pixel at the click point using the Robot class’ getPixelColor method. It then converts the pixel’s color to a CSS-style color format (RGBA) and sets the background color of the application window to the selected color, providing a real-time color feedback effect for the clicked pixel.
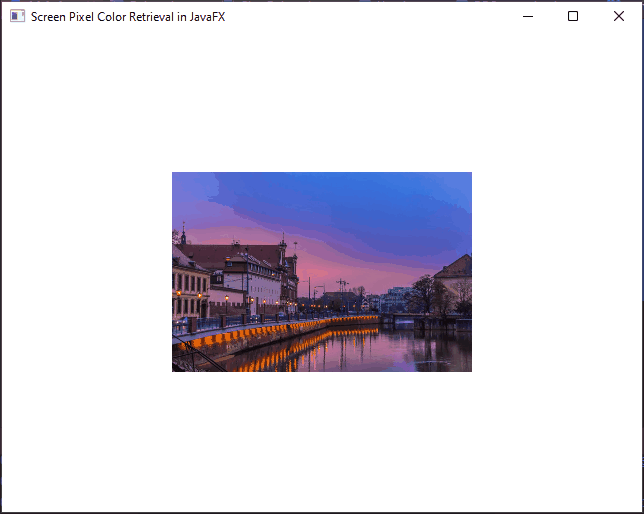
Use Cases for Screen Pixel Color Retrieval
There are various scenarios where screen pixel color retrieval can be beneficial:
- Color Picker: You can build a custom color picker tool that allows users to select colors from anywhere on the screen.
- Screen Capture: When capturing a specific region of the screen, you may need to know the color of individual pixels to apply various effects.
- Automated Testing: In automated testing of JavaFX applications, you can verify that the colors displayed on the screen match expected values.
- Accessibility: You can use pixel color information to implement accessibility features such as color contrast analysis.
- Image Processing: When working with image processing applications, knowing the colors of pixels is essential for various algorithms.
Conclusion
The Robot class in JavaFX provides a powerful way to interact with the screen, including retrieving pixel colors. This feature can be used in a wide range of applications, from color pickers to automated testing and image processing. By putting into use the capabilities of the Robot class, you can create more dynamic and powerful JavaFX applications that go beyond the standard user interface components.
Additional Resources:
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter.