JavaFX is a versatile and powerful framework for building interactive and visually appealing user interfaces in Java applications. One of its key features is the Canvas API, which allows developers to draw graphics and render text directly onto a canvas. In this article, we’ll focus on working with text in a JavaFX Canvas.
Rendering Text on a Canvas
Rendering text on a Canvas involves using the fillText() and strokeText() methods provided by the GraphicsContext class. These methods allow you to specify the text content, position, font, and color.
Rendering Stroked Text
To render stroked text on the canvas, you can use the strokeText method. Here’s an example of how to use it:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
import javafx.scene.text.FontWeight;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(600, 400);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set font, stroke color, and stroke width
gc.setFont(Font.font("Arial", FontWeight.BOLD, 24));
gc.setStroke(Color.RED);
gc.setLineWidth(2.0);
// Draw stroked text
gc.strokeText("Hello, JavaFX!", 50, 100);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Text in JavaFX Canvas: Adding Typography to Your Graphics");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this example, we draw stroked text on the canvas. We set the font, stroke color, and stroke width using the setFont(), setStroke(), and setLineWidth() methods, and then use strokeText() to render the text at coordinates (50, 100).
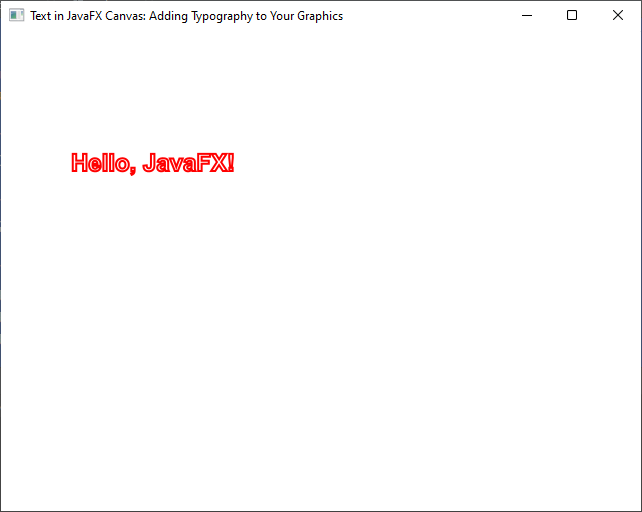
Rendering Filled Text
To render filled (solid) text on the canvas, you can use the fillText method:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
import javafx.scene.text.FontWeight;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(600, 400);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set font, and fill color
gc.setFont(Font.font("Arial", FontWeight.BOLD, 24));
gc.setFill(Color.BLUE);
// Draw filled text
gc.fillText("Hello, JavaFX!", 50, 100);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Text in JavaFX Canvas: Adding Typography to Your Graphics");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this example, we create a simple JavaFX application that displays filled text on a canvas. We set the font, fill color, and position (50, 100) of the text using the setFont(), setFill(), and fillText() methods.
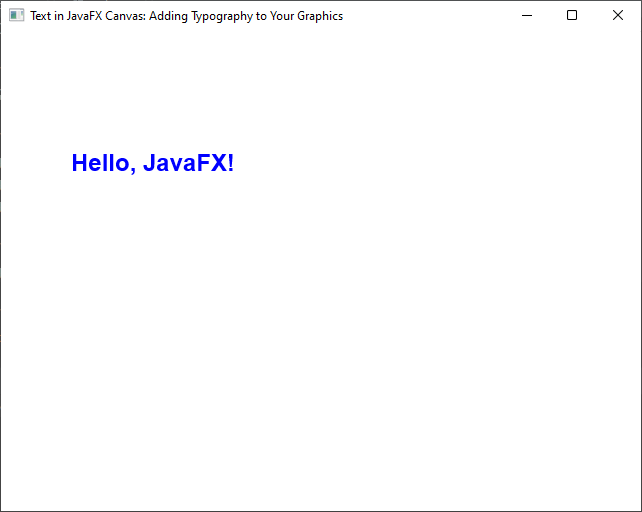
Controlling Text Width
In some cases, you may want to limit the width of the rendered text, especially when dealing with dynamic content or text that needs to fit within a specific area. The GraphicsContext class provides overloaded versions of strokeText and fillText that accept a maxWidth parameter. This parameter allows you to specify the maximum width the text can occupy on the canvas.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
import javafx.scene.text.FontWeight;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(600, 400);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set font, fill color
gc.setFont(Font.font("Arial", FontWeight.BOLD, 24));
// Set stroke color
gc.setStroke(Color.RED);
// Set fill color
gc.setFill(Color.BLUE);
// Set stroke width
gc.setLineWidth(2.0);
// Draw text with maxWidth
gc.strokeText("Lorem ipsum dolor sit amet, consectetur adipiscing elit.", 50, 50, 200);
gc.fillText("Lorem ipsum dolor sit amet, consectetur adipiscing elit.", 50, 100, 200);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Text in JavaFX Canvas: Adding Typography to Your Graphics");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this example, the text will be rendered within a maximum width of 200 pixels.
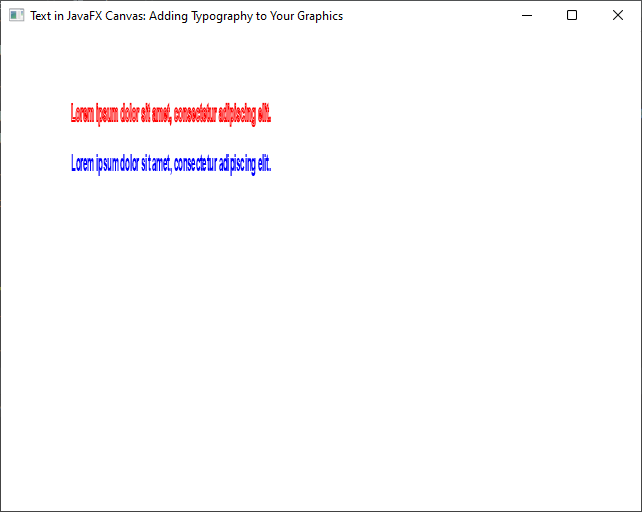
Text Alignment
Horizontal Text Alignment
In JavaFX, text alignment plays a pivotal role in fine-tuning the appearance of your graphical elements. The setTextAlign() method is your go-to tool for controlling horizontal alignment when rendering text on a JavaFX canvas. This method provides you with the ability to ensure your text is precisely positioned in relation to the specified coordinates.
The setTextAlign() method takes a single argument, align, which belongs to the TextAlignment enumeration. This enumeration offers three distinct values:
- TextAlignment.LEFT: Aligns the text to the left of the specified coordinates.
- TextAlignment.CENTER: Centers the text horizontally around the specified coordinates.
- TextAlignment.RIGHT: Aligns the text to the right of the specified coordinates.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
import javafx.scene.text.FontWeight;
import javafx.scene.text.TextAlignment;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(600, 400);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set font, stroke color, and stroke width
gc.setFont(Font.font("Arial", FontWeight.BOLD, 24));
gc.setStroke(Color.RED);
gc.setLineWidth(2.0);
// Draw a line as a visual cue
gc.strokeLine(50, 0, 50, 250);
// Draw stroked text (LEFT aligned)
gc.strokeText("Hello, JavaFX!", 50, 100);
// Centers the text horizontally
gc.setTextAlign(TextAlignment.CENTER);
// Draw stroked text, (CENTER aligned)
gc.strokeText("Hello, JavaFX!", 50, 150);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Text in JavaFX Canvas: Adding Typography to Your Graphics");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this example, we set the text alignment to TextAlignment.CENTER. As a result, the text will be perfectly centered horizontally around the specified coordinates when rendered on the canvas.
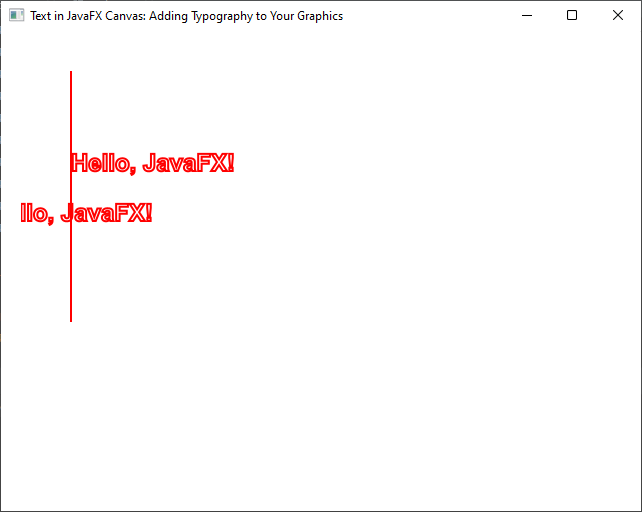
Vertical Text Alignment
Vertical alignment of text is just as important as horizontal alignment, especially when crafting visually pleasing graphics and interfaces in JavaFX. To manage vertical alignment, we turn to the setTextBaseline() method.
setTextBaseline() is your tool for controlling the vertical positioning of text when drawing on a JavaFX canvas. This method accepts a single argument, baseline, which belongs to the VPos enumeration. The available values for baseline include:
- VPos.BASELINE: Aligns the text with its baseline at the specified vertical position.
- VPos.TOP: Aligns the text with its top at the specified vertical position.
- VPos.CENTER: Centers the text vertically around the specified position.
- VPos.BOTTOM: Aligns the text with its bottom at the specified vertical position.
import javafx.application.Application;
import javafx.geometry.VPos;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
import javafx.scene.text.FontWeight;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(600, 400);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set font, stroke color, and stroke width
gc.setFont(Font.font("Arial", FontWeight.BOLD, 24));
gc.setStroke(Color.RED);
gc.setLineWidth(2.0);
// Draw lines as visual cues
gc.strokeLine(50, 0, 50, 100);
gc.strokeLine(300, 0, 300, 100);
gc.strokeLine(50, 300, 50, 200);
gc.strokeLine(300, 300, 300, 200);
// Draw stroked text
gc.strokeText("Hello, BASELINE!", 50, 100);
// Set text baseline to VPos.TOP
gc.setTextBaseline(VPos.TOP);
// Draw stroked text
gc.strokeText("Hello, TOP!", 300, 100);
// Set text baseline to VPos.CENTER
gc.setTextBaseline(VPos.CENTER);
// Draw stroked text
gc.strokeText("Hello, CENTER!", 50, 300);
// Set text baseline to VPos.BOTTOM
gc.setTextBaseline(VPos.BOTTOM);
// Draw stroked text
gc.strokeText("Hello, BOTTOM!", 300, 300);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Text in JavaFX Canvas: Adding Typography to Your Graphics");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
In this example, we demonstrated the effect of various vertical alignments (VPos) on text positioning by drawing lines as visual cues and text at different positions on the canvas. With each text element, the vertical alignment is adjusted using gc.setTextBaseline(VPosā¦), resulting in the text being displayed either at the top, center, or bottom of the specified coordinates. This example provides a hands-on illustration of how to control the vertical alignment of text within JavaFX graphics, allowing for precise and visually appealing text placement.
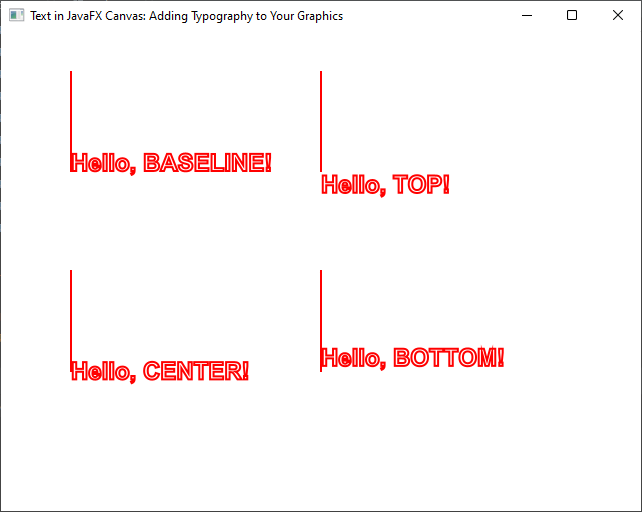
Fine-Tuning Text Position with Both Methods
To achieve pixel-perfect text placement, it’s often necessary to combine both setTextAlign() and setTextBaseline() methods. This combination enables you to precisely control both horizontal and vertical text alignment, resulting in beautifully composed graphics.
Consider a scenario where you want to align text to the top-right corner of a specified point on the canvas:
import javafx.application.Application;
import javafx.geometry.VPos;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
import javafx.scene.text.FontWeight;
import javafx.scene.text.TextAlignment;
import javafx.stage.Stage;
public class Main extends Application {
private final BorderPane parent = new BorderPane();
@Override
public void init() throws Exception {
super.init();
// Build the user interface
this.buildUI();
}
private void buildUI() {
// Create the Canvas
Canvas canvas = new Canvas(600, 400);
// Get the GraphicsContext
GraphicsContext gc = canvas.getGraphicsContext2D();
// Set font, stroke color, and stroke width
gc.setFont(Font.font("Arial", FontWeight.BOLD, 24));
gc.setStroke(Color.RED);
gc.setLineWidth(2.0);
// Draw lines as visual cues
gc.strokeLine(220, 0, 220, 100);
// Set text alignment to right and text baseline to top
gc.setTextAlign(TextAlignment.RIGHT);
gc.setTextBaseline(VPos.TOP);
// Draw stroked text with the specified alignment
gc.strokeText("Hello, TOP RIGHT!", 220, 100);
// Add the Canvas to the center of the BorderPane
this.parent.setCenter(canvas);
}
@Override
public void start(Stage stage) throws Exception {
// Setup and display the stage
this.setupStage(stage);
}
private void setupStage(Stage stage) {
// Create a scene with the BorderPane as the root
Scene scene = new Scene(this.parent, 640, 480);
// Set the stage title
stage.setTitle("Text in JavaFX Canvas: Adding Typography to Your Graphics");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Display the stage
stage.show();
}
}
By setting the horizontal alignment to TextAlignment.RIGHT and the vertical alignment to VPos.TOP, you can achieve this specific text placement, creating a visually pleasing composition.
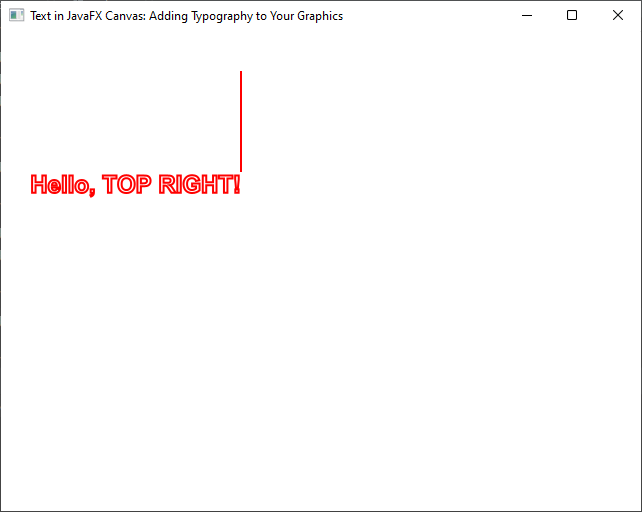
Conclusion
In JavaFX, rendering text on a Canvas is made straightforward with the strokeText and fillText methods. These methods allow you to draw text in various styles and colors, and by using the maxWidth parameter, you can control the width of the text, making it adaptable to different layouts and requirements within your JavaFX applications. This flexibility empowers you to create visually appealing and dynamic user interfaces with ease.
By mastering text rendering in JavaFX, you’ll be better equipped to design engaging and informative graphical applications that effectively convey information to users. Remember to check the JavaFX Canvas Documentation for more.
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter!