JavaFX is a versatile framework for building interactive and visually appealing user interfaces. One of its many features is the ability to apply various visual effects to UI elements, adding a layer of dynamism and user engagement to your applications. In this article, we’ll explore the ImageInput effect, a fascinating tool that allows you to incorporate external images into your JavaFX applications without altering the original image itself.
What is the ImageInput Effect?
The ImageInput effect, as the name suggests, is a JavaFX effect used to pass a specified image as input to another effect. Unlike other effects, when you apply the ImageInput effect to a node, it won’t modify the original image. Instead, it serves as an input source for other effects, allowing you to combine multiple effects to achieve the desired visual result in your application.
Creating ImageInput Effect Example Application
To understand the ImageInput Effect better, let’s dive into a practical example where we use JavaFX to apply this effect in a Java application. In this example, we’ll use ImageInput to pass an image as input to the SepiaTone effect, resulting in a visually appealing transformation of a simple rectangle.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.effect.ImageInput;
import javafx.scene.effect.SepiaTone;
import javafx.scene.image.Image;
import javafx.scene.layout.BorderPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
public class ImageInputEffect extends Application {
// Application properties
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
// Create the main application scene
Scene scene = new Scene(this.parent, WIDTH, HEIGHT);
// Set the title for the application window
stage.setTitle("ImageInput Effect");
// Set the scene for the stage
stage.setScene(scene);
// Center the stage on the screen
stage.centerOnScreen();
// Show the stage on the screen
stage.show();
}
@Override
public void init() throws Exception {
super.init();
// Create a Rectangle Node
Rectangle rectangle = new Rectangle(300, 300);
rectangle.setFill(Color.CORNFLOWERBLUE); // Set the Rectangle Fill color
// Create the ImageInput Effect
Image image = new Image("rose.jpg"); // Load an image
ImageInput imageInput = new ImageInput(image);
// Adjust the position of the displayed image
imageInput.setX(0);
imageInput.setY(0);
// Create the SepiaTone Effect
SepiaTone sepiaTone = new SepiaTone();
// Set the ImageInput as the input effect to the SepiaTone Effect
sepiaTone.setInput(imageInput);
// Apply the SepiaTone Effect to the Rectangle
rectangle.setEffect(sepiaTone);
// Add the Rectangle to the center region of the BorderPane
this.parent.setCenter(rectangle);
}
}
In this example, we start by creating a blue rectangle using JavaFX’s Rectangle class. We then load an image called “rose.jpg” using the Image class. The ImageInput effect is created with this image as input, and we position it at the coordinates (0,0) within the node.
Next, we create a SepiaTone effect and set the ImageInput effect as its input. This causes the SepiaTone effect to be applied to the image, resulting in a sepia-toned version of the image. Finally, we set the SepiaTone effect as the effect applied to the blue rectangle, and add the rectangle to the center of the BorderPane.
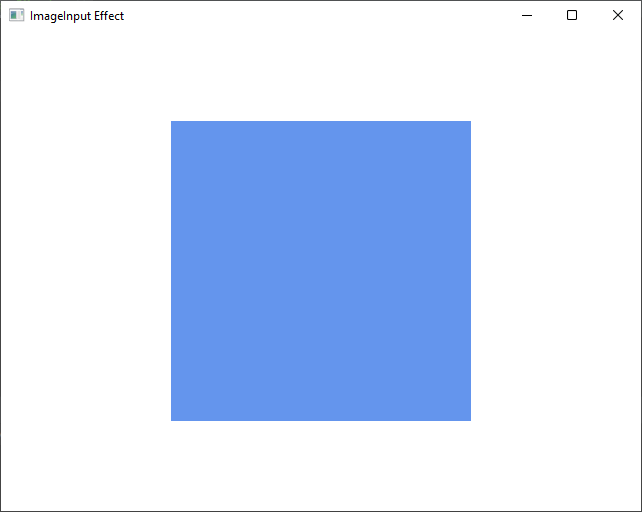
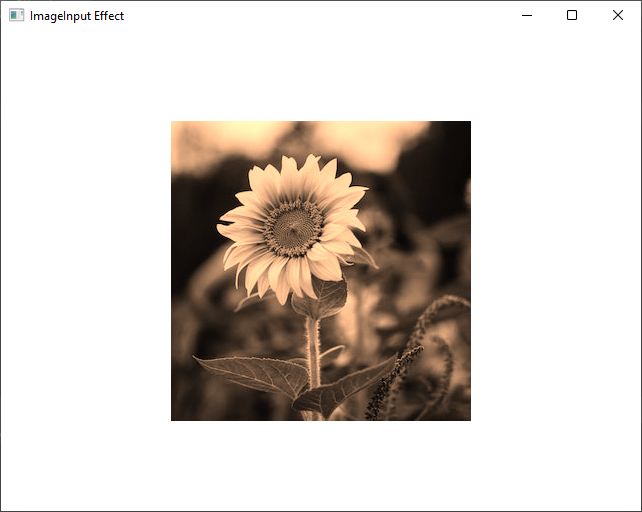
The image on the left is before the effect is applied to the rectangle, and the one on the right is after the effect is applied to the rectangle.
Conclusion
In conclusion, the JavaFX ImageInput Effect proves to be an invaluable tool in the world of graphic rendering and visual effects. As we’ve explored in this article, the ImageInput effect plays a unique role, allowing you to pass an unmodified image as an input for other effects, opening up a realm of creative possibilities.
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter.