Handling international phone numbers in applications can be challenging, and standard text fields often fall short in providing the necessary features for accurate formatting and validation. Recognizing this limitation, the PhoneNumberField leverages the robust capabilities of the Google libphonenumber library. This library, known for its excellence in parsing, formatting, and validating international phone numbers, forms the foundation of the PhoneNumberField. In this article, we’ll explore the features of the PhoneNumberField and how it simplifies the handling of phone numbers in your JavaFX applications.
Introduction
The PhoneNumberField control is designed to alleviate the complexities associated with entering phone numbers in JavaFX applications. Leveraging the power of the Google libphonenumber library, this control streamlines the process of formatting, validating, and selecting the appropriate country code.
Features of PhoneNumberField
Country Selection Dropdown
At the core of the PhoneNumberField is a dynamic dropdown for selecting the country, empowering users to easily choose their country for accurate parsing and validation of phone numbers.
Auto-selection of Country Based on Country Code
A noteworthy feature of the PhoneNumberField is its ability to automatically select the country based on the provided country code. As users input their phone number, the control intelligently detects the country code and adjusts the selected country accordingly.
Automatic Formatting
Another standout feature of the PhoneNumberField is its real-time formatting as users type their phone number. This feature ensures a consistent and visually pleasing representation of phone numbers.
Validation
Beyond formatting, the control actively validates entered phone numbers based on the selected country. The dynamic validation state keeps users informed about the correctness of their input, enhancing data accuracy.
Preferred Countries
To cater to individual user preferences, the PhoneNumberField allows the prioritization of preferred countries, customizing the user experience by placing commonly used countries at the top of the dropdown list.
Installation
To integrate the PhoneNumberField into your JavaFX application, you need to add the following Maven dependency assuming you are using maven. At the time of this writing, the most recent version available was 1.9.0:
<dependency>
<groupId>com.dlsc.phonenumberfx</groupId>
<artifactId>phonenumberfx</artifactId>
<version>1.9.0</version>
</dependency>
This dependency ensures that your project includes the necessary PhoneNumberField library. For more details and updates, refer to the official GitHub repository: PhoneNumberFX GitHub Repo.
Creating a Basic PhoneNumberField
Integrating the PhoneNumberField into your JavaFX application is as straightforward as incorporating any other control. Simply instantiate the PhoneNumberField and add it to your layout:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
import com.dlsc.phonenumberfx.PhoneNumberField;
public class BasicPhoneNumberInput extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
Scene scene = new Scene(this.parent, WIDTH, HEIGHT);
// Set the stage title
stage.setTitle("JavaFX: Phone Number Input Field");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create an instance of PhoneNumberField
PhoneNumberField phoneNumberField = new PhoneNumberField();
// Set the PhoneNumberField max width to 200.0px
phoneNumberField.setMaxWidth(200.0);
// Add the PhoneNumberField to the center of the BorderPane
parent.setCenter(phoneNumberField);
}
}
With these few lines of code, you’ve now equipped your application with a sophisticated phone number input field. The PhoneNumberField will automatically format the entered phone number based on the selected country and validate it in real-time.
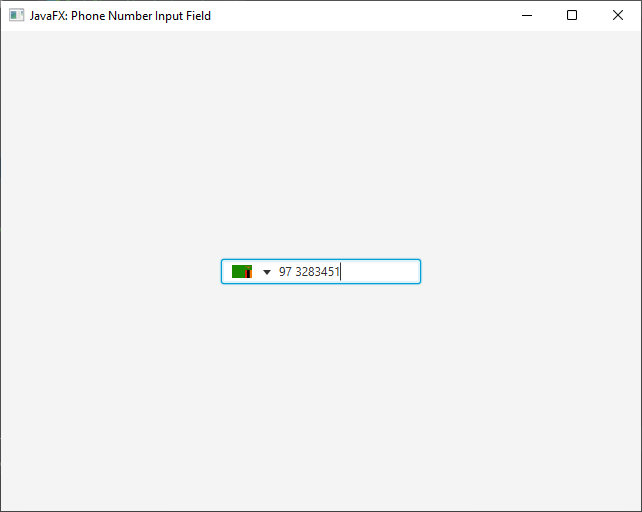
Setting the Selected Country
The PhoneNumberField allows users to select their country, ensuring accurate formatting and validation. You can also programmatically set the selected country based on your application’s requirements.
Programmatic Selection
To programmatically set the selected country, use the setSelectedCountry method:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
import com.dlsc.phonenumberfx.PhoneNumberField;
public class SelectCountryExample extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
Scene scene = new Scene(this.parent, WIDTH, HEIGHT);
// Set the stage title
stage.setTitle("JavaFX: Phone Number Input Field - Setting Selected Country");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create an instance of PhoneNumberField
PhoneNumberField phoneNumberField = new PhoneNumberField();
// Set the PhoneNumberField max width to 200.0px
phoneNumberField.setMaxWidth(200.0);
// Set the selected country to Zambia
phoneNumberField.setSelectedCountry(PhoneNumberField.Country.ZAMBIA);
// Add the PhoneNumberField to the center of the BorderPane
parent.setCenter(phoneNumberField);
}
}
This code example demonstrates how to set the selected country to Zambia. Adjust the country according to your application’s requirements.
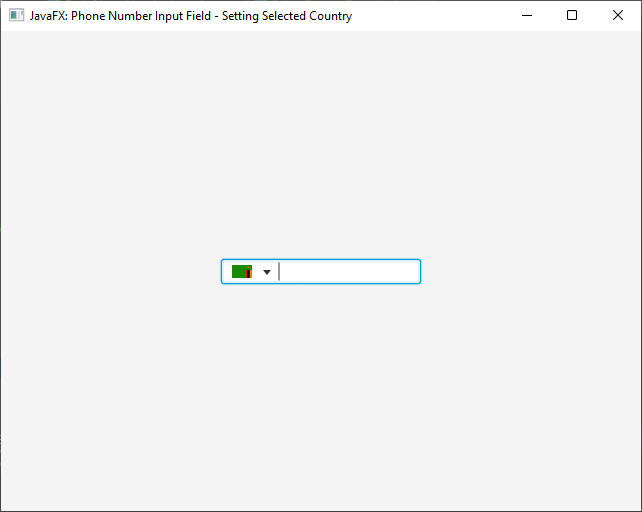
Dynamic Country Resolving
The control intelligently resolves the country based on the entered phone number. As users type, the control dynamically updates the selected country.
Specifying Expected Phone Number Type
The setExpectedPhoneNumberType method in the PhoneNumberField control allows developers to define the expected type of phone numbers that are considered valid for their application. This feature is crucial for enforcing specific constraints on the accepted phone number formats, ensuring a more controlled and accurate input.
Available Phone Number Types
The PhoneNumberField accommodates a variety of phone number types, each serving a distinct purpose. Here are some notable phone number types that can be used with the setExpectedPhoneNumberType method:
- PhoneNumberType.FIXED_LINE: Denotes fixed-line phone numbers typically associated with residences or businesses.
- PhoneNumberType.MOBILE: Represents mobile phone numbers commonly assigned to individuals.
- PhoneNumberType.FIXED_LINE_OR_MOBILE: Encompasses both fixed-line and mobile phone numbers.
- PhoneNumberType.TOLL_FREE: Signifies toll-free phone numbers often used for customer service lines.
- PhoneNumberType.PREMIUM_RATE: Identifies premium rate phone numbers associated with services incurring additional charges.
- PhoneNumberType.SHARED_COST: Indicates shared-cost phone numbers where the caller and recipient share the expense.
- PhoneNumberType.VOIP: Represents Voice over Internet Protocol (VoIP) phone numbers relying on internet connectivity.
- PhoneNumberType.PERSONAL_NUMBER: Denotes personal phone numbers.
- PhoneNumberType.PAGER: Identifies pager numbers, although less common in contemporary usage.
- PhoneNumberType.UAN: Stands for Universal Access Numbers.
- PhoneNumberType.VOICEMAIL: Denotes voicemail access numbers.
- PhoneNumberType.UNKNOWN: A placeholder for an unknown phone number type.
Consider the following example program:
import com.dlsc.phonenumberfx.PhoneNumberField;
import com.google.i18n.phonenumbers.PhoneNumberUtil;
import javafx.application.Application;
import javafx.beans.value.ObservableValue;
import javafx.collections.FXCollections;
import javafx.event.ActionEvent;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.CheckBox;
import javafx.scene.control.ComboBox;
import javafx.scene.control.Label;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class PhoneNumberTypeExample extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
Scene scene = new Scene(this.parent, WIDTH, HEIGHT);
// Set the stage title
stage.setTitle("JavaFX: Phone Number Input Field - Phone Number Type");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
@Override
public void init() throws Exception {
super.init();
buildUI();
}
private void buildUI() {
BasicForm basicForm = new BasicForm();
// Add the BasicForm to the center of the BorderPane
parent.setCenter(basicForm);
}
/**
* A form demonstrating the usage of PhoneNumberField with expected phone number types.
*/
static class BasicForm extends VBox {
private final PhoneNumberField phoneNumberField;
private final Label labelValidity;
public BasicForm() {
// Phone number types list
PhoneNumberUtil.PhoneNumberType[] phoneNumberTypes = PhoneNumberUtil.PhoneNumberType.values();
ComboBox<PhoneNumberUtil.PhoneNumberType> phoneNumberTypeComboBox =
new ComboBox<>(FXCollections.observableArrayList(phoneNumberTypes));
// Create an instance of PhoneNumberField
this.phoneNumberField = new PhoneNumberField();
this.phoneNumberField.expectedPhoneNumberTypeProperty().bind(phoneNumberTypeComboBox.valueProperty());
// Create a show example number CheckBox
CheckBox showExampleNumberCheckBox = new CheckBox("Show Example Number");
showExampleNumberCheckBox.setSelected(this.phoneNumberField.isShowExampleNumbers());
showExampleNumberCheckBox.selectedProperty().addListener(this::onShowExampleNumber);
// Create a Label to show example phone numbers
Label labelExample = new Label();
labelExample.textProperty().bind(this.phoneNumberField.promptTextProperty());
// Create a Button to submit entered phone number
Button button = new Button("Submit");
button.setOnAction(this::onSubmit);
// Create a Label to show the validity of the number
this.labelValidity = new Label();
// Add controls to the VBox container
VBox container = new VBox(
15.0,
new VBox(
5.0,
this.phoneNumberField,
labelExample
),
phoneNumberTypeComboBox,
showExampleNumberCheckBox,
button,
this.labelValidity
);
// Set the VBox container max width
container.setMaxWidth(500.0);
this.setAlignment(Pos.CENTER);
this.getChildren().addAll(container);
}
private void onSubmit(ActionEvent actionEvent) {
if (this.phoneNumberField.isValid()) {
labelValidity.setText("The entered phone number is valid.");
labelValidity.setStyle("-fx-text-fill: green;");
} else {
labelValidity.setText("The entered phone number is not valid.");
labelValidity.setStyle("-fx-text-fill: red;");
}
}
private void onShowExampleNumber(ObservableValue<? extends Boolean> observableValue, Boolean oldValue, Boolean newValue) {
phoneNumberField.showExampleNumbersProperty().set(newValue);
}
}
}
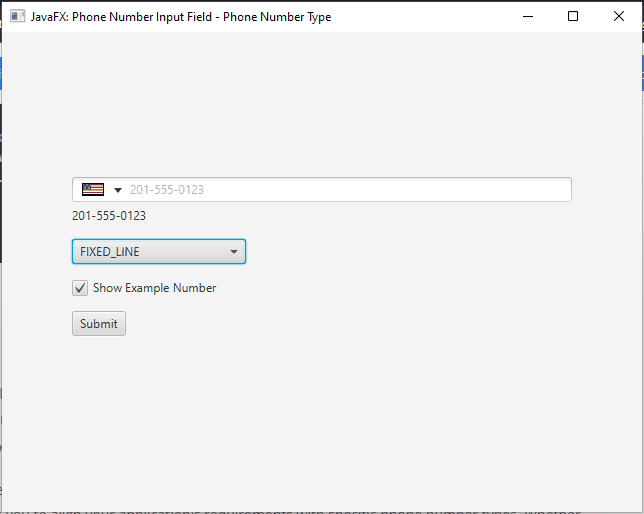
This example provides a functional application where users can interact with the PhoneNumberField, set the expected phone number type, and validate entered phone numbers. The PhoneNumberField dynamically adjusts the displayed example number based on the selected phone number type. Additionally, it provides feedback on the validity of the entered phone number.
The setExpectedPhoneNumberType method in the PhoneNumberField class not only adds a layer of precision to your phone number input validation but also allows you to align your application’s requirements with specific phone number types. Whether you’re validating mobile numbers, toll-free lines, or a combination thereof, this method provides the flexibility needed to enhance the accuracy and integrity of phone number input in your applications.
Displaying Example Numbers
Enabling the showExampleNumbers (setShowExampleNumbers(true)) property in the PhoneNumberField control triggers the display of example numbers as prompt text. The feature serves as a visual guide for users, showing them the expected format of the phone number based on the selected country. It’s important to note that this feature is presented as prompt text and remains visible only for empty fields, and is only visible when the field does not have focus.
import javafx.application.Application;
import javafx.beans.value.ObservableValue;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.CheckBox;
import javafx.scene.control.Label;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
import com.dlsc.phonenumberfx.PhoneNumberField;
public class ShowExampleNumberExample extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
Scene scene = new Scene(this.parent, WIDTH, HEIGHT);
// Set the stage title
stage.setTitle("JavaFX: Phone Number Input Field - Show Example Number");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
BasicForm basicForm = new BasicForm();
// Add the BasicForm to the center of the BorderPane
parent.setCenter(basicForm);
}
static class BasicForm extends VBox {
private final PhoneNumberField phoneNumberField;
public BasicForm() {
// Create an instance of PhoneNumberField
this.phoneNumberField = new PhoneNumberField();
// Create a show example number CheckBox
CheckBox showExampleNumber = new CheckBox("Show Example Number");
showExampleNumber.selectedProperty().addListener(this::onShowExampleNumber);
// Create a Label to show example phone numbers
Label labelExample = new Label();
labelExample.textProperty().bind(this.phoneNumberField.promptTextProperty());
// Add the PhoneNumberField,
// Button, and the CheckBox to the VBox container
VBox container = new VBox(
15.0,
this.phoneNumberField,
labelExample,
showExampleNumber
);
// Set the VBox container max width
container.setMaxWidth(200.0);
this.setAlignment(Pos.CENTER);
this.getChildren().addAll(container);
}
private void onShowExampleNumber(ObservableValue<? extends Boolean> observableValue, Boolean oldValue, Boolean newValue) {
this.phoneNumberField.showExampleNumbersProperty().set(newValue);
}
}
}
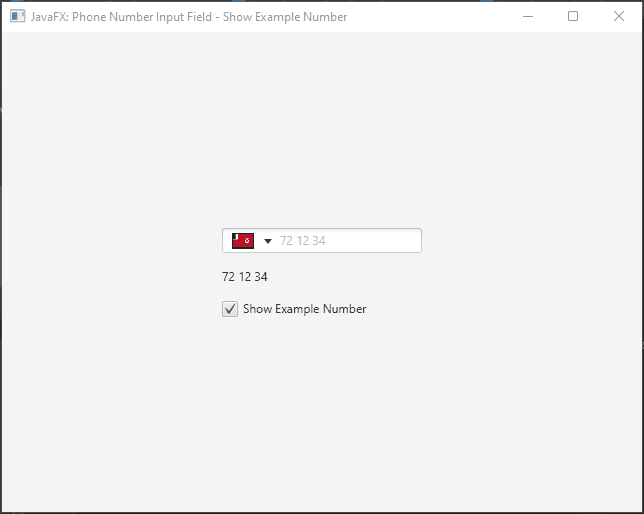
When users interact with the PhoneNumberField, they will see a dynamically changing prompt text that mirrors the expected phone number format for the selected country. This provides users with a clear understanding of how to input their phone numbers correctly. For instance, if a user selects the United States as the country, the prompt text might elegantly demonstrate an example like “e.g., 555-123-4567.”
Country Code Visibility
The setCountryCodeVisible method offers developers the flexibility to control the visibility of the country code within the PhoneNumberField control. When set to true, the country code is displayed, providing users with a clear indication of the selected country’s code. Conversely, setting it to false hides the country code, offering a more streamlined appearance.
import com.dlsc.phonenumberfx.PhoneNumberField;
import javafx.application.Application;
import javafx.beans.value.ObservableValue;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.CheckBox;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class ShowCountryCodeExample extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
Scene scene = new Scene(this.parent, WIDTH, HEIGHT);
// Set the stage title
stage.setTitle("JavaFX: Phone Number Input Field - Country Code Visibility");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
BasicForm basicForm = new BasicForm();
// Add the BasicForm to the center of the BorderPane
parent.setCenter(basicForm);
}
static class BasicForm extends VBox {
private final PhoneNumberField phoneNumberField;
public BasicForm() {
// Create an instance of PhoneNumberField
this.phoneNumberField = new PhoneNumberField();
// Create a show country code CheckBox
CheckBox showCountryCodeCheckBox = new CheckBox("Show Country Code");
showCountryCodeCheckBox.setSelected(this.phoneNumberField.isCountryCodeVisible());
showCountryCodeCheckBox.selectedProperty().addListener(this::onShowCountryCode);
// Add controls to the VBox container
VBox container = new VBox(
15.0,
this.phoneNumberField,
showCountryCodeCheckBox
);
// Set the VBox container max width
container.setMaxWidth(200.0);
this.setAlignment(Pos.CENTER);
this.getChildren().addAll(container);
}
private void onShowCountryCode(ObservableValue<? extends Boolean> observableValue, Boolean oldValue, Boolean newValue) {
this.phoneNumberField.countryCodeVisibleProperty().set(newValue);
}
}
}
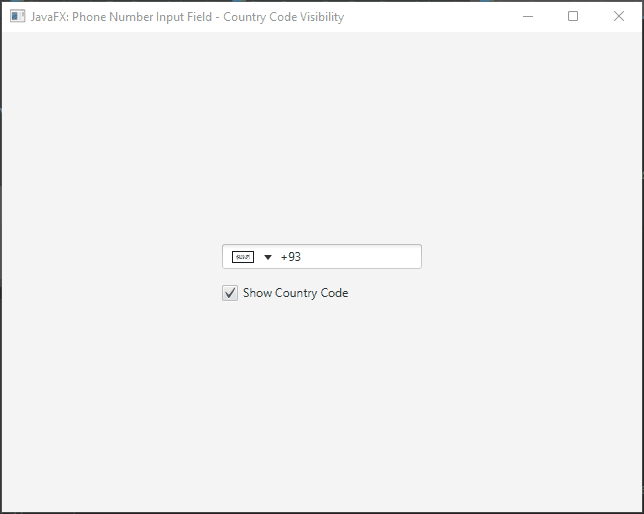
In this example, invoking setCountryCodeVisible(true) ensures that the country code is visible alongside the phone number input. This can be beneficial in scenarios where users need to verify the selected country code.
Getting Available Countries
If you need to access the list of available countries in the PhoneNumberField, you can use the getAvailableCountries() method:
import com.dlsc.phonenumberfx.PhoneNumberField;
import javafx.application.Application;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.control.ListView;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
public class GetAvailableCountriesExample extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
Scene scene = new Scene(this.parent, WIDTH, HEIGHT);
// Set the stage title
stage.setTitle("JavaFX: Phone Number Input Field - Getting Available Countries");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create an instance of PhoneNumberField
PhoneNumberField phoneNumberField = new PhoneNumberField();
// Set the PhoneNumberField max width to 200.0px
phoneNumberField.setMaxWidth(200.0);
// Retrieve the list of available countries
ObservableList<PhoneNumberField.Country> availableCountries = phoneNumberField.getAvailableCountries();
ListView<PhoneNumberField.Country> countryListView = new ListView<>(availableCountries);
// Add the PhoneNumberField to the top of the BorderPane
parent.setTop(phoneNumberField);
// Add the Country ListView to the center of the BorderPane
parent.setCenter(countryListView);
}
}
This provides you with the flexibility to iterate over the available countries, display them in your UI, or perform any other custom logic.
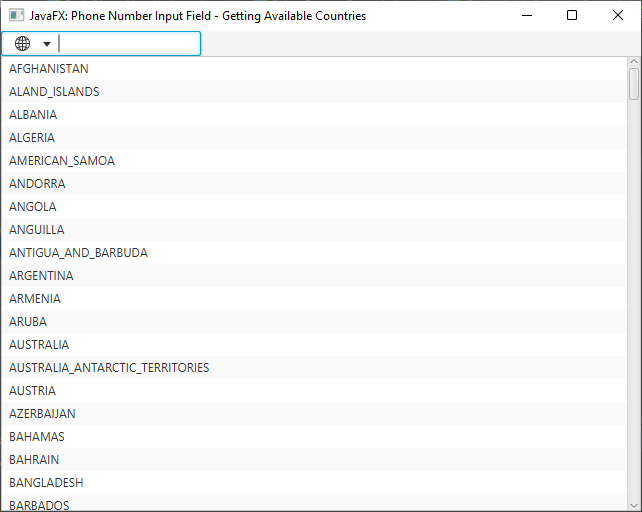
Setting Preferred Countries
The PhoneNumberField allows users to prioritize certain countries for a personalized experience. Developers can preconfigure a list of preferred countries to suit the target user base. Preferred countries appear at the top of the country selection dropdown, providing users with quick access to the most relevant options. Here’s how you can set up preferred countries:
import com.dlsc.phonenumberfx.PhoneNumberField;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
import java.util.Arrays;
import java.util.List;
public class PreferredCountriesExample extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
Scene scene = new Scene(this.parent, WIDTH, HEIGHT);
// Set the stage title
stage.setTitle("JavaFX: Phone Number Input Field - Preferred Countries");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create an instance of PhoneNumberField
PhoneNumberField phoneNumberField = new PhoneNumberField();
// Set the PhoneNumberField max width to 200.0px
phoneNumberField.setMaxWidth(200.0);
// Define a list of preferred countries
List<PhoneNumberField.Country> preferredCountries = Arrays.asList(
PhoneNumberField.Country.CANADA,
PhoneNumberField.Country.UNITED_KINGDOM,
PhoneNumberField.Country.UNITED_STATES,
PhoneNumberField.Country.ZAMBIA
);
// Set the preferred countries for the PhoneNumberField
phoneNumberField.getPreferredCountries().setAll(preferredCountries);
// Add the PhoneNumberField to the center of the BorderPane
parent.setCenter(phoneNumberField);
}
}
In this example, the preferredCountries list includes Canada, the United Kingdom, the United States, and Zambia. Adjust the list to reflect the countries most relevant to your application. By adding countries to the list of preferred countries, you influence the order in which they appear in the dropdown. Users can easily access commonly used countries, streamlining the selection process.
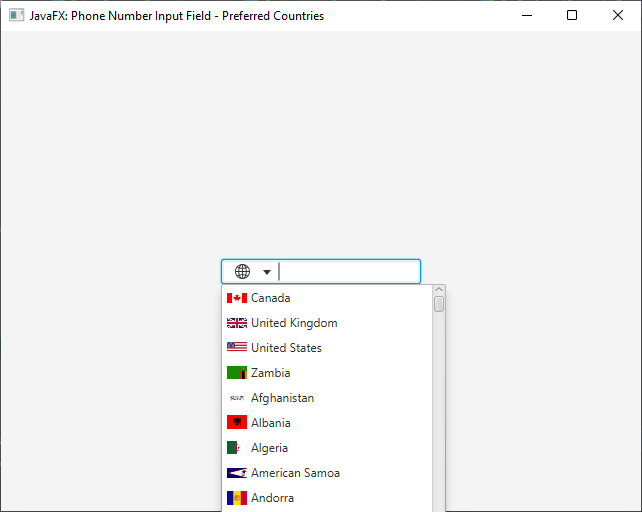
Getting Preferred Countries
To retrieve the list of preferred countries from the PhoneNumberField, you can use the getPreferredCountries() method:
import com.dlsc.phonenumberfx.PhoneNumberField;
import javafx.application.Application;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.control.ListView;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
public class GetPreferredCountriesExample extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
Scene scene = new Scene(this.parent, WIDTH, HEIGHT);
// Set the stage title
stage.setTitle("JavaFX: Phone Number Input Field - Getting Preferred Countries");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create an instance of PhoneNumberField
PhoneNumberField phoneNumberField = new PhoneNumberField();
// Set the PhoneNumberField max width to 200.0px
phoneNumberField.setMaxWidth(200.0);
// Set the preferred countries for the PhoneNumberField
phoneNumberField.getPreferredCountries().setAll(
PhoneNumberField.Country.CANADA,
PhoneNumberField.Country.UNITED_KINGDOM,
PhoneNumberField.Country.UNITED_STATES,
PhoneNumberField.Country.ZAMBIA
);
// Retrieve the list of preferred countries
ObservableList<PhoneNumberField.Country> preferredCountries = phoneNumberField.getPreferredCountries();
ListView<PhoneNumberField.Country> countryListView = new ListView<>(preferredCountries);
// Add the PhoneNumberField to the top of the BorderPane
parent.setTop(phoneNumberField);
// Add the Country ListView to the center of the BorderPane
parent.setCenter(countryListView);
}
}
By accessing the list of preferred countries, you can dynamically adjust your application’s logic based on the countries prioritized in the PhoneNumberField dropdown.
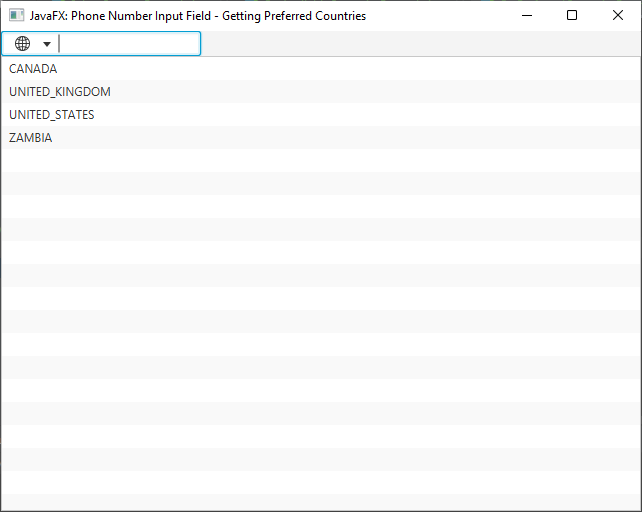
Validation State
Determining the validity of the entered phone number is a crucial aspect of data integrity. The PhoneNumberField provides a convenient method for this purpose, the isValid method():
import com.dlsc.phonenumberfx.PhoneNumberField;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.VBox;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class ValidationState extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
Scene scene = new Scene(this.parent, WIDTH, HEIGHT);
// Set the stage title
stage.setTitle("JavaFX: Phone Number Input Field - Validation State");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
BasicForm basicForm = new BasicForm();
// Add the Basic to the center of the BorderPane
parent.setCenter(basicForm);
}
static class BasicForm extends VBox {
private final PhoneNumberField phoneNumberField;
private final Label label;
public BasicForm() {
// Create an instance of PhoneNumberField
this.phoneNumberField = new PhoneNumberField();
// Create a submit Button
Button button = new Button("Submit");
button.setOnAction(this::onSubmit);
// Create a result label
this.label = new Label();
// Add the PhoneNumberField, Button, and the Label to the VBox container
VBox container = new VBox(
10.0,
this.phoneNumberField,
button,
this.label
);
// Set the VBox container max width
container.setMaxWidth(200.0);
this.setAlignment(Pos.CENTER);
this.getChildren().addAll(container);
}
public void onSubmit(ActionEvent event) {
if(this.phoneNumberField.isValid()) {
this.label.setText("Entered phone number is valid!");
this.label.setTextFill(Color.GREEN);
} else {
this.label.setText("Entered phone number is invalid.");
this.label.setTextFill(Color.RED);
}
}
}
}
The isValid() method returns a boolean value indicating whether the currently entered phone number is valid according to the selected country’s format and rules. This feature enables you to provide real-time feedback to users and implement additional actions based on the validity of the entered phone number.
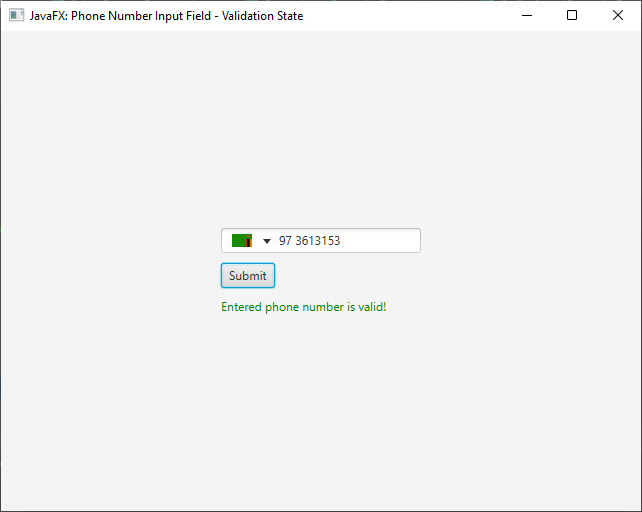
Accessing Phone Number Information
In addition to validation, the PhoneNumberField facilitates the retrieval of essential phone number details, with the getRawPhoneNumber(), getNationalPhoneNumber() and getE164PhoneNumber() methods:
import com.dlsc.phonenumberfx.PhoneNumberField;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class PhoneNumberInformation extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
Scene scene = new Scene(this.parent, WIDTH, HEIGHT);
// Set the stage title
stage.setTitle("JavaFX: Phone Number Input Field - Phone Number Information");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
BasicForm basicForm = new BasicForm();
// Add the Basic to the center of the BorderPane
parent.setCenter(basicForm);
}
static class BasicForm extends VBox {
private final PhoneNumberField phoneNumberField;
private final Label rawPhoneNumberLabel;
private final Label nationalPhoneNumberLabel;
private final Label e164PhoneNumberLabel;
public BasicForm() {
// Create an instance of PhoneNumberField
this.phoneNumberField = new PhoneNumberField();
// Create a submit Button
Button button = new Button("Submit");
button.setOnAction(this::onSubmit);
// Create a rawPhoneNumber label
this.rawPhoneNumberLabel = new Label();
// Create a nationalPhoneNumber label
this.nationalPhoneNumberLabel = new Label();
// Create a e164PhoneNumber label
this.e164PhoneNumberLabel = new Label();
// Add the PhoneNumberField,
// Button, and the Labels to the VBox container
VBox container = new VBox(
10.0,
this.phoneNumberField,
button,
this.rawPhoneNumberLabel,
this.nationalPhoneNumberLabel,
this.e164PhoneNumberLabel
);
// Set the VBox container max width
container.setMaxWidth(200.0);
this.setAlignment(Pos.CENTER);
this.getChildren().addAll(container);
}
public void onSubmit(ActionEvent event) {
if(this.phoneNumberField.isValid()) {
// Retrieve the raw phone number
String rawPhoneNumber = phoneNumberField.getRawPhoneNumber();
this.rawPhoneNumberLabel.setText("Raw Phone Number: " + rawPhoneNumber);
// Retrieve the national formatted number
String nationalPhoneNumber = phoneNumberField.getNationalPhoneNumber();
this.nationalPhoneNumberLabel.setText("National Phone Number: " + nationalPhoneNumber);
// Retrieve the E.164 formatted number
String e164PhoneNumber = phoneNumberField.getE164PhoneNumber();
this.e164PhoneNumberLabel.setText("E164 Phone Number: " + e164PhoneNumber);
}
}
}
}
These methods provide developers with access to the raw, national, and E.164 formatted versions of the entered phone number. This information can be valuable for various purposes, such as backend processing, storage, or displaying formatted information to users.
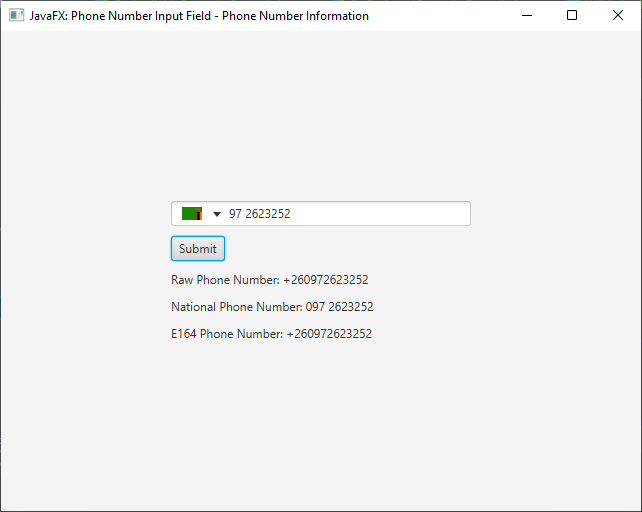
Getting the Selected Country
To retrieve the currently selected country from the PhoneNumberField, you can use the getSelectedCountry() method:
import com.dlsc.phonenumberfx.PhoneNumberField;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
import java.util.Arrays;
public class GetSelectedCountryExample extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
Scene scene = new Scene(this.parent, WIDTH, HEIGHT);
// Set the stage title
stage.setTitle("JavaFX: Phone Number Input Field - Get Selected Country");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
BasicForm basicForm = new BasicForm();
// Add the Basic to the center of the BorderPane
parent.setCenter(basicForm);
}
static class BasicForm extends VBox {
private final PhoneNumberField phoneNumberField;
private final Label selectedCountryLabel;
public BasicForm() {
// Create an instance of PhoneNumberField
this.phoneNumberField = new PhoneNumberField();
// Create a submit Button
Button button = new Button("Submit");
button.setOnAction(this::onSubmit);
// Create a selectedCountry label
this.selectedCountryLabel = new Label();
// Add the PhoneNumberField,
// Button, and the selectedCountry Label to the VBox container
VBox container = new VBox(
10.0,
this.phoneNumberField,
button,
this.selectedCountryLabel
);
// Set the VBox container max width
container.setMaxWidth(200.0);
this.setAlignment(Pos.CENTER);
this.getChildren().addAll(container);
}
public void onSubmit(ActionEvent event) {
if(this.phoneNumberField.isValid()) {
// Retrieve the selected country
PhoneNumberField.Country country = phoneNumberField.getSelectedCountry();
// Display the selected country
this.selectedCountryLabel.setText(
"NAME: " + country.name() +
"\nCOUNTRY CODE: " + country.countryCode() +
"\nCOUNTRY CODE PREFIX: " + country.countryCodePrefix() +
"\nISO2 CODE: " + country.iso2Code() +
"\nAREA CODES: " + Arrays.toString(country.areaCodes()) +
"\nDEFAULT AREA CODES: " + country.defaultAreaCode()
);
}
}
}
}
This method returns the Country object representing the currently selected country. Developers can use this information to perform additional actions or validations based on the selected country.
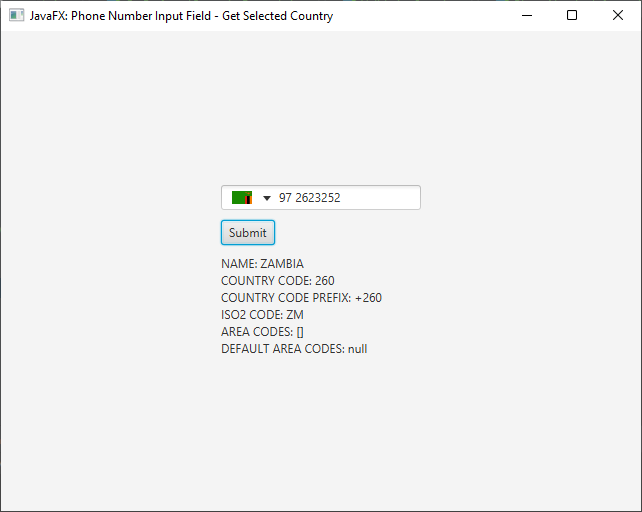
Clearing the PhoneNumberField
Clearing the entered phone number in the PhoneNumberField is a common requirement, especially in scenarios where users need to start fresh or remove a previously entered number. The PhoneNumberField provides a simple method to achieve this, the clear() method:
import com.dlsc.phonenumberfx.PhoneNumberField;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class ClearPhoneNumberInput extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
Scene scene = new Scene(this.parent, WIDTH, HEIGHT);
// Set the stage title
stage.setTitle("JavaFX: Phone Number Input Field - Clear");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
BasicForm basicForm = new BasicForm();
// Add the Basic to the center of the BorderPane
parent.setCenter(basicForm);
}
static class BasicForm extends VBox {
private final PhoneNumberField phoneNumberField;
public BasicForm() {
// Create an instance of PhoneNumberField
this.phoneNumberField = new PhoneNumberField();
// Create a submit Button
Button submitButton = new Button("Submit");
submitButton.setOnAction(this::onSubmit);
// Create a clear Button
Button clearButton = new Button("Clear");
clearButton.setOnAction(this::onClear);
// Add the PhoneNumberField,
// Submit and Clear Buttons to the VBox container
VBox container = new VBox(
10.0,
this.phoneNumberField,
new HBox(5, submitButton, clearButton)
);
// Set the VBox container max width
container.setMaxWidth(200.0);
this.setAlignment(Pos.CENTER);
this.getChildren().addAll(container);
}
private void onClear(ActionEvent actionEvent) {
// Clear the PhoneNumberField
this.phoneNumberField.clear();
}
public void onSubmit(ActionEvent event) {}
}
}
By invoking the clear method on the PhoneNumberField instance, you effectively remove the entered phone number and reset the control to its initial state. This action can be triggered in response to user interactions or as part of a broader functionality within your JavaFX application.
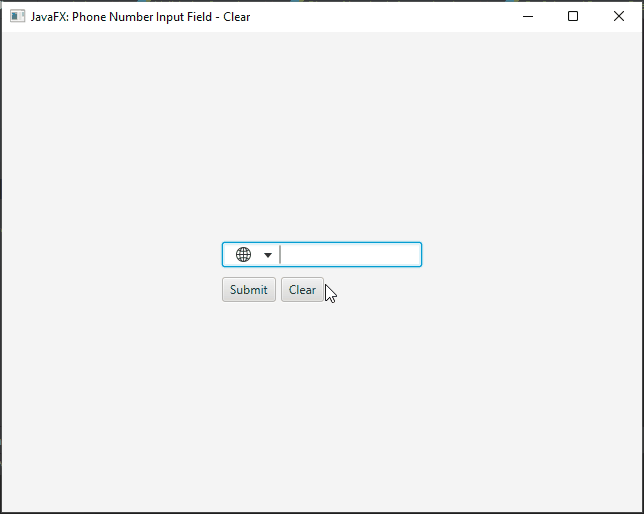
Disabling the PhoneNumberField
There might be scenarios where you want to disable user input in the PhoneNumberField. Here’s how you can achieve that:
import com.dlsc.phonenumberfx.PhoneNumberField;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
public class DisablePhoneNumberInput extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
Scene scene = new Scene(this.parent, WIDTH, HEIGHT);
// Set the stage title
stage.setTitle("JavaFX: Phone Number Input Field - Disable");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create an instance of PhoneNumberField
PhoneNumberField phoneNumberField = new PhoneNumberField();
// Set the PhoneNumberField max width to 200.0px
phoneNumberField.setMaxWidth(200.0);
// Disable the PhoneNumberField
phoneNumberField.setDisable(true);
// Add the PhoneNumberField to the center of the BorderPane
parent.setCenter(phoneNumberField);
}
}
By calling the setDisable method with the value true, you effectively disable user input in the PhoneNumberField. This can be useful in situations where phone number input is not currently required or allowed.
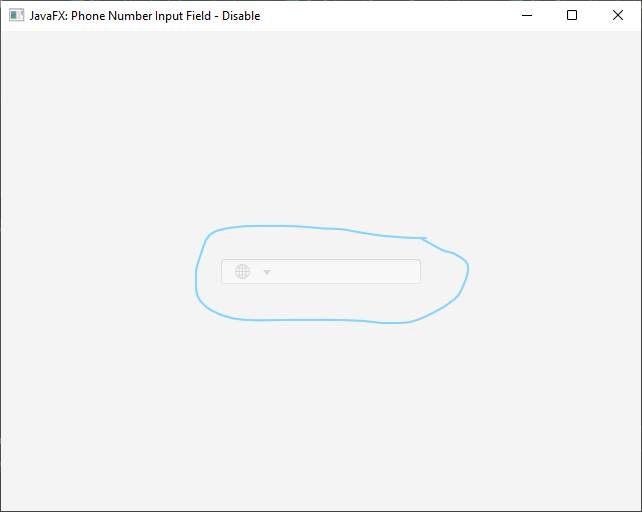
Disabling the PhoneNumberField Dropdown Only
If you prefer to disable only the dropdown functionality of the PhoneNumberField, leaving the input field enabled, you can use the following:
import com.dlsc.phonenumberfx.PhoneNumberField;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
public class DisablePhoneNumberInputDropDown extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
Scene scene = new Scene(this.parent, WIDTH, HEIGHT);
// Set the stage title
stage.setTitle("JavaFX: Phone Number Input Field - Disable DropDown");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
// Create an instance of PhoneNumberField
PhoneNumberField phoneNumberField = new PhoneNumberField();
// Set the PhoneNumberField max width to 200.0px
phoneNumberField.setMaxWidth(200.0);
// Set the selected country to Zambia
phoneNumberField.setSelectedCountry(PhoneNumberField.Country.ZAMBIA);
// Disable the PhoneNumberField DropDown
phoneNumberField.setDisableCountryDropdown(true);
// Add the PhoneNumberField to the center of the BorderPane
parent.setCenter(phoneNumberField);
}
}
This allows users to view and interact with the phone number input field while preventing them from selecting a different country from the dropdown.
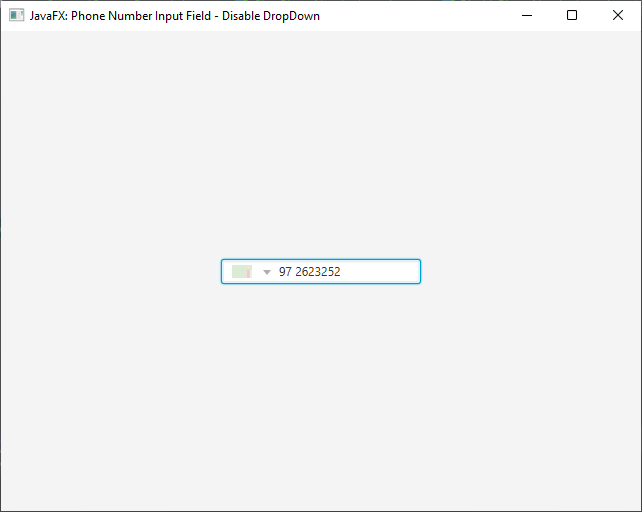
Show or Hide the Country Dropdown
The setShowCountryDropdown method plays a pivotal role in determining the visibility of the country dropdown within the PhoneNumberField control. When set to true, the dropdown is displayed, allowing users to select their country of residence. Conversely, setting it to false hides the dropdown, providing a more compact interface.
import com.dlsc.phonenumberfx.PhoneNumberField;
import javafx.application.Application;
import javafx.beans.value.ObservableValue;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.CheckBox;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class ShowCountryDropDownExample extends Application {
private static final double WIDTH = 640;
private static final double HEIGHT = 480;
private final BorderPane parent = new BorderPane();
@Override
public void start(Stage stage) throws Exception {
Scene scene = new Scene(this.parent, WIDTH, HEIGHT);
// Set the stage title
stage.setTitle("JavaFX: Phone Number Input Field - CountryDropDown Visibility");
// Sets the stage scene
stage.setScene(scene);
// Centers stage on screen
stage.centerOnScreen();
// Show stage on screen
stage.show();
}
@Override
public void init() throws Exception {
super.init();
this.buildUI();
}
private void buildUI() {
BasicForm basicForm = new BasicForm();
// Add the BasicForm to the center of the BorderPane
parent.setCenter(basicForm);
}
static class BasicForm extends VBox {
private final PhoneNumberField phoneNumberField;
public BasicForm() {
// Create an instance of PhoneNumberField
this.phoneNumberField = new PhoneNumberField();
// Create a show country drop down CheckBox
CheckBox showCountryDropdownCheckBox = new CheckBox("Show Country Dropdown");
showCountryDropdownCheckBox.setSelected(this.phoneNumberField.isShowCountryDropdown());
showCountryDropdownCheckBox.selectedProperty().addListener(this::onShowCountryDropDown);
// Add controls to the VBox container
VBox container = new VBox(
15.0,
this.phoneNumberField,
showCountryDropdownCheckBox
);
// Set the VBox container max width
container.setMaxWidth(200.0);
this.setAlignment(Pos.CENTER);
this.getChildren().addAll(container);
}
private void onShowCountryDropDown(ObservableValue<? extends Boolean> observableValue, Boolean oldValue, Boolean newValue) {
this.phoneNumberField.showCountryDropdownProperty().set(newValue);
}
}
}
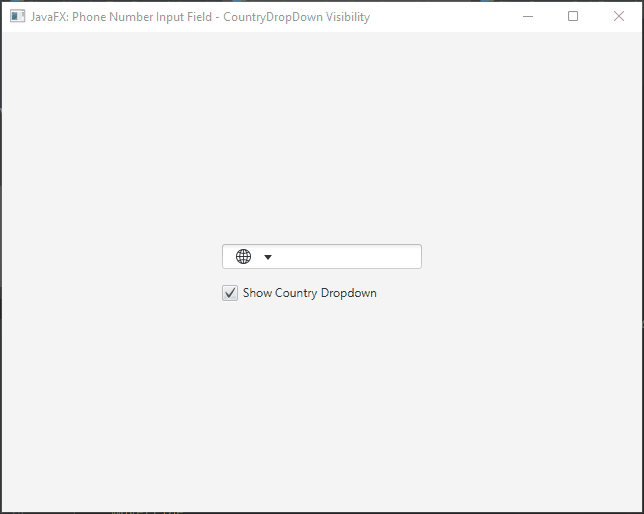
By invoking the setShowCountryDropdown method with the argument true, the country dropdown becomes visible, enabling users to interactively choose their country. This dynamic interaction enhances the user experience, especially in applications where users may have varying country codes.
Styling and Customization
The PhoneNumberField provides ample opportunities for styling and customization using CSS. Developers can tailor the appearance of the control to align with the application’s design.
/* Sample CSS for styling PhoneNumberField */
.phone-number-field {
-fx-background-color: #d2f3f3; /* Set background color */
-fx-padding: 10px; /* Set padding */
-fx-border-color: cornflowerblue; /* set border-color */
}
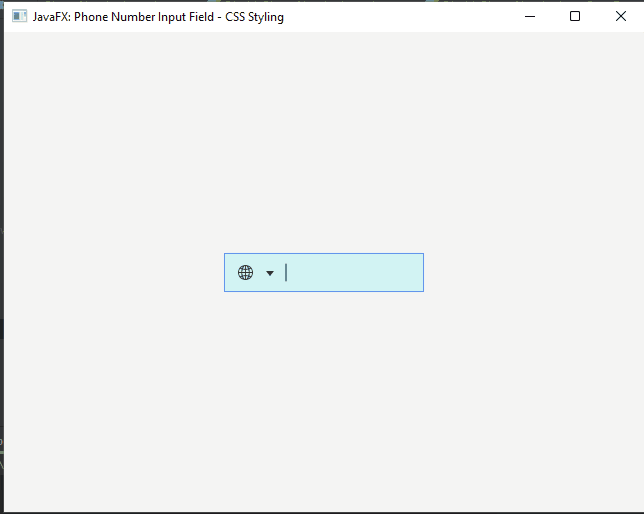
Conclusion
In conclusion, the PhoneNumberField presents itself as a powerful solution for handling phone numbers in JavaFX applications. By seamlessly integrating the Google libphonenumber library, the control ensures accurate formatting, validation, and an overall enhanced user experience. The added feature of auto-selection based on the country code further simplifies the input process. As software developers, embracing specialized input fields like the PhoneNumberField is pivotal in delivering applications that prioritize user convenience and data accuracy.
I hope you found this article informative and useful. If you would like to receive more content, please consider subscribing to our newsletter.